Retool Email
You can send emails to your users from Retool apps and workflows. You can use Retool Email, a built-in SMTP service, or add your own as a resource.
An example of where this might be useful is if you are using these dashboards for customer service. You can have an automated email sent to the player if they are banned or un-banned or if customer support has added virtual-currency to the player in order to resolve a support case.
In this example we will be using emails to send promotional codes to the player which could be redeemed in-app for a reward.
On the ‘Players’ tab, create a new modal frame and name it ‘emailModal’, then click on the table, add a row-action called ‘Email’ and set the icon to ‘email’. Add an event handler to the action to open the modal.
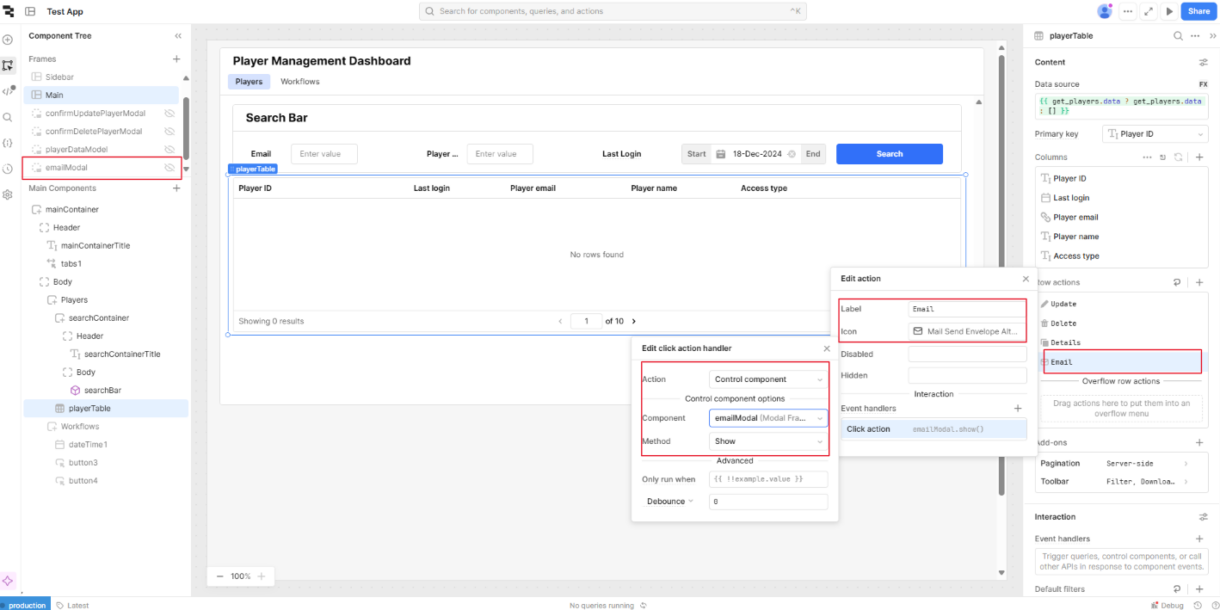
After this, set up the modal components by adding two text input fields and a text area. Update the labels and give the components the following names:
emailAddress
emailSubject
emailBody
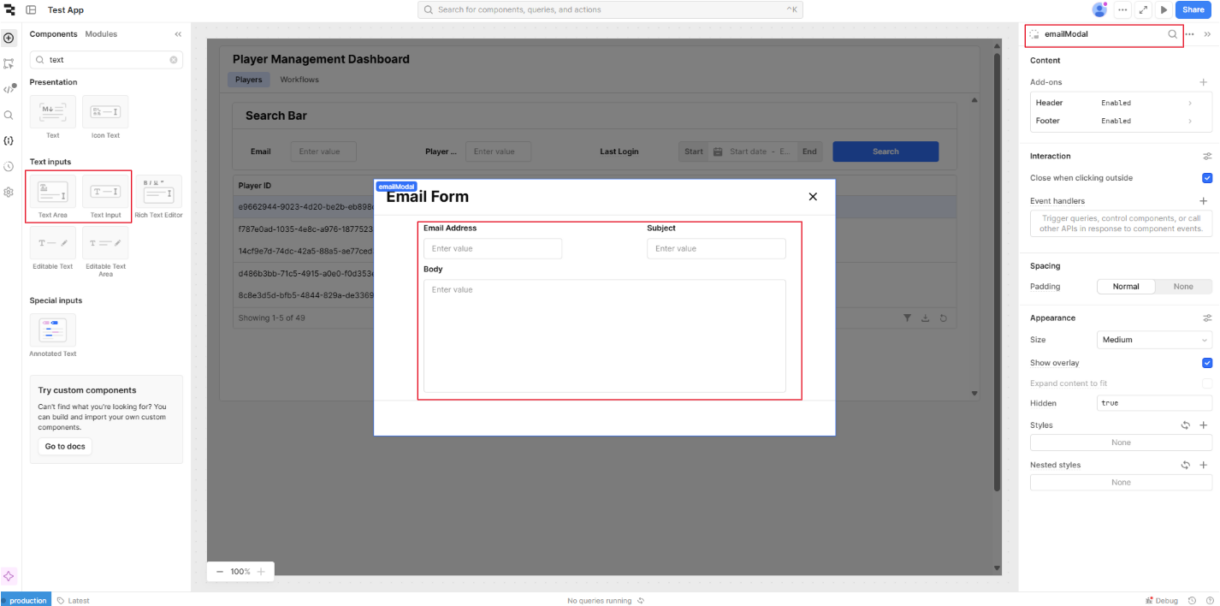
We will use 3 Retool queries to set up and send the email.
- JavaScript query called ‘email_promo_code’ to generate the promotion code.
const characters = 'ABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789';
const randomChars = Array.from({ length: 6 }, () => characters[Math.floor(Math.random() * characters.length)]);
const code = randomChars.slice(0, 3).join('') + '-' + randomChars.slice(3).join('');
return code;
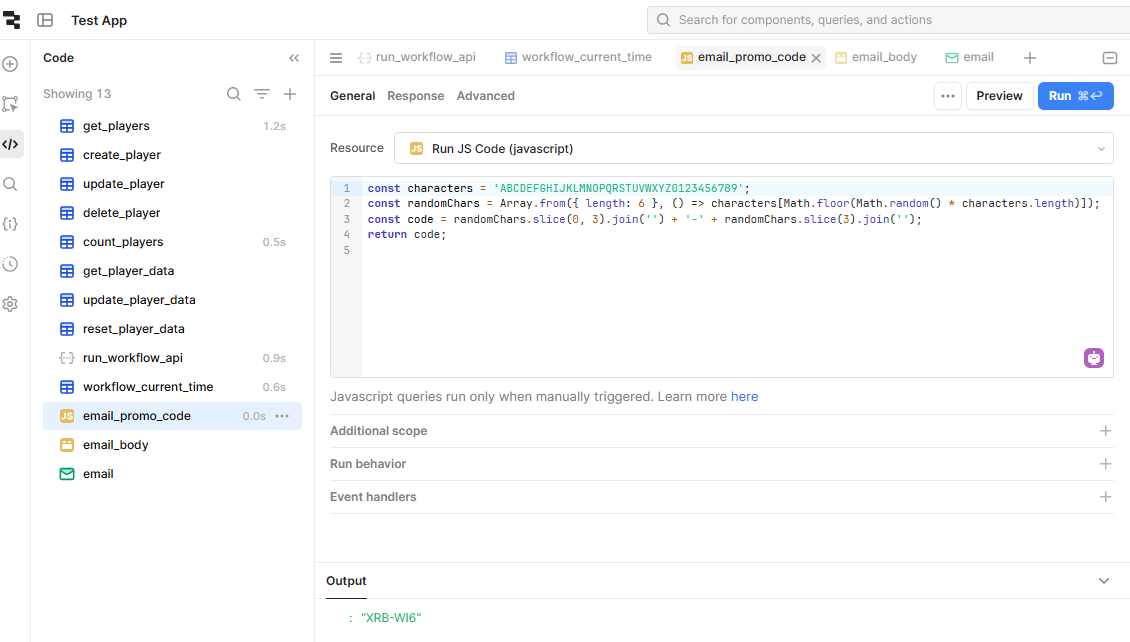
2. JavaScript transformer ‘email_body’ to format the email body.
const name = {{ playerTable.selectedRow.full_name }}
const code = {{email_promo_code.data}};
const string = `Congratulations ${name}!\n\nYou have been selected to receive a promotion code to enhance your gaming experience.\n\n
Use the following code to unlock new items or missions or upgrade existing items in your inventory.\n
${code}\n\nHappy Gaming!\n\nTeam SuperNimbus`;
return string;
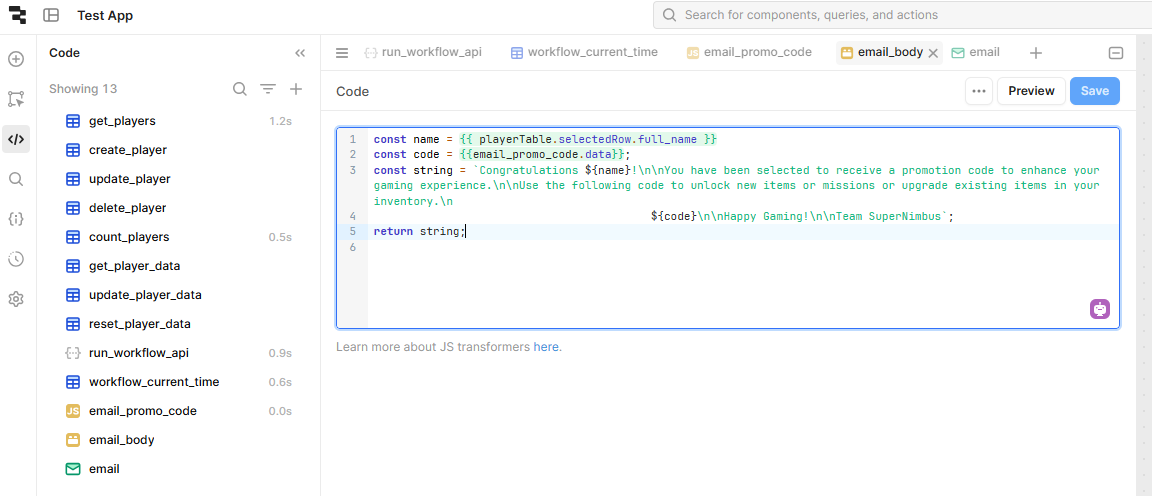
Retool Resource Email query ‘email’ to send the email, using the following references.
{{ emailAddress.value }}
{{ emailSubject.value }}
{{ emailBody.value }}
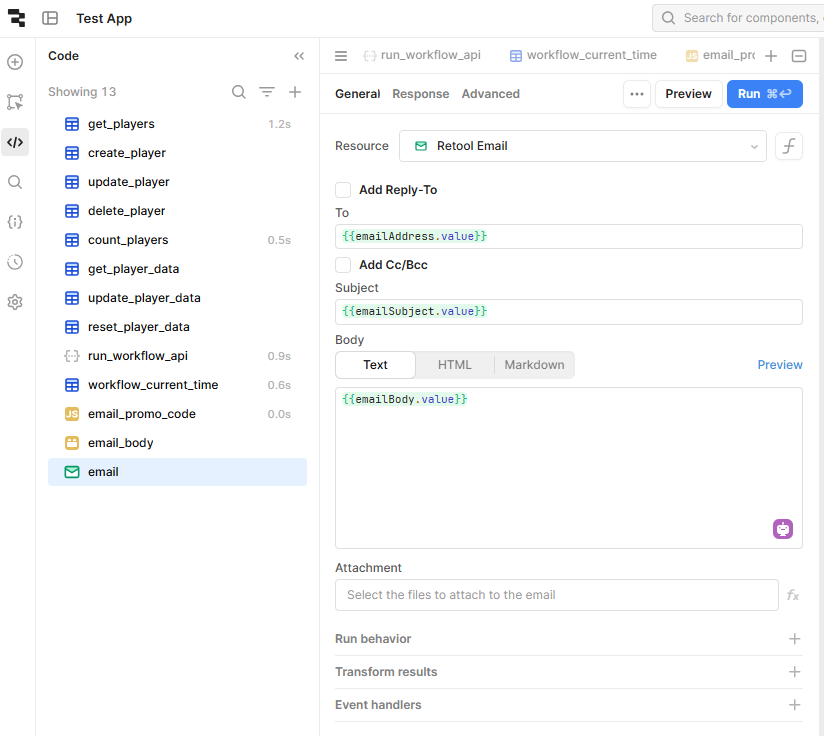
Set up the email form button in the modal linked to the ‘email’ query.
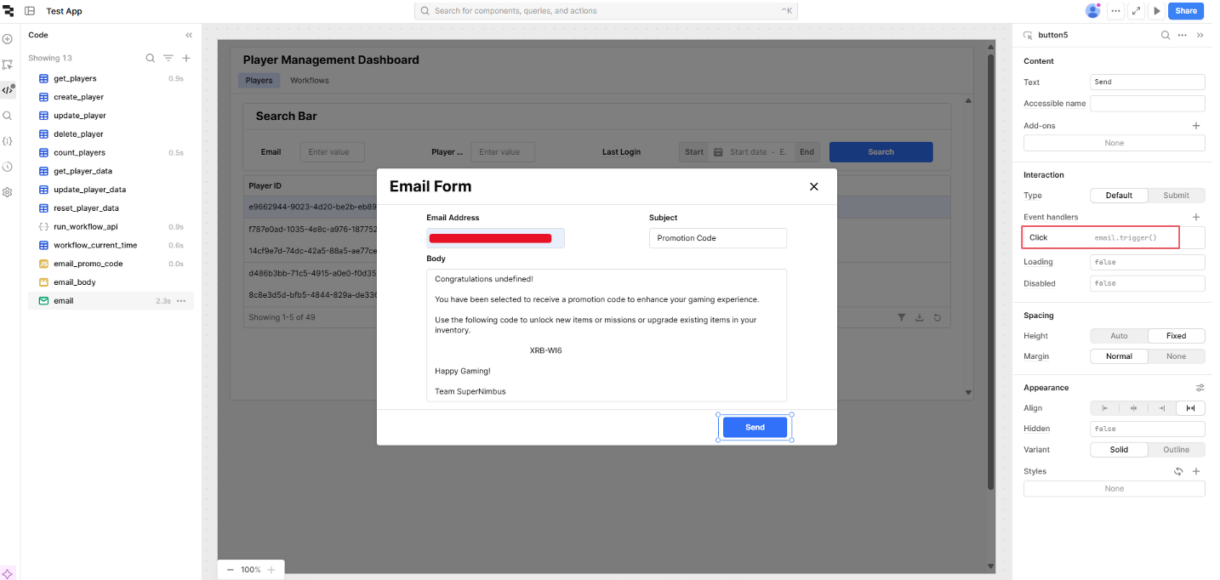
Set the ‘emailAddress’ text field to reference the email address of the selected row. This can be made ‘read-only’ after it has been tested.
{{ playerTable.data[0].player_email }}
Set the body section to reference the transformer value.
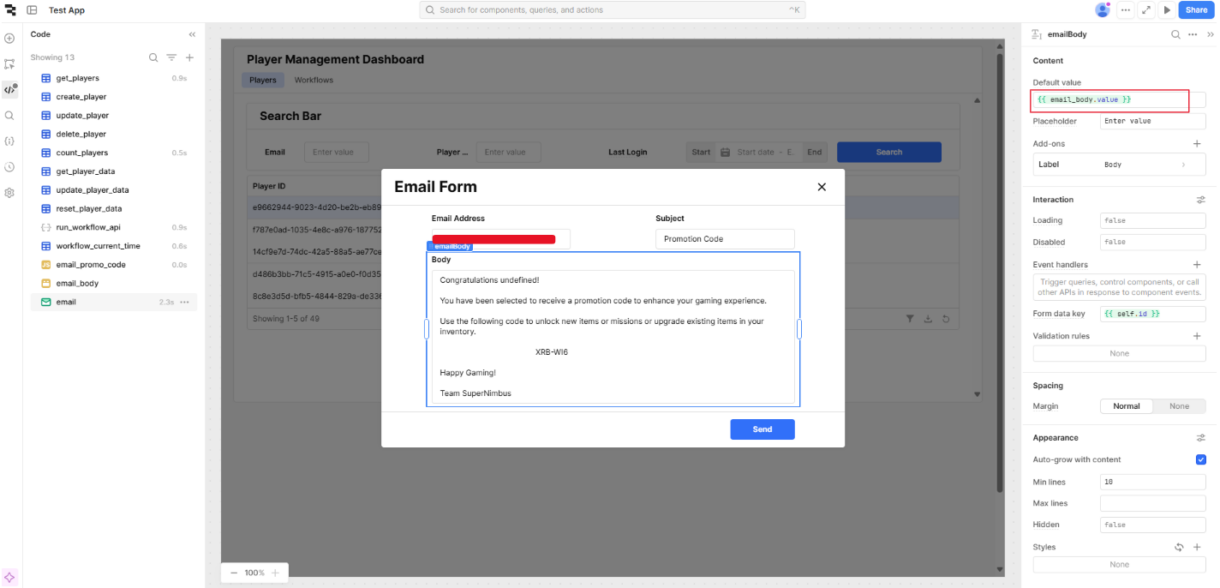
{{ email_body.value }}
Add the Retool ‘confetti’ utility function as a success event, indicating that the email has been sent.
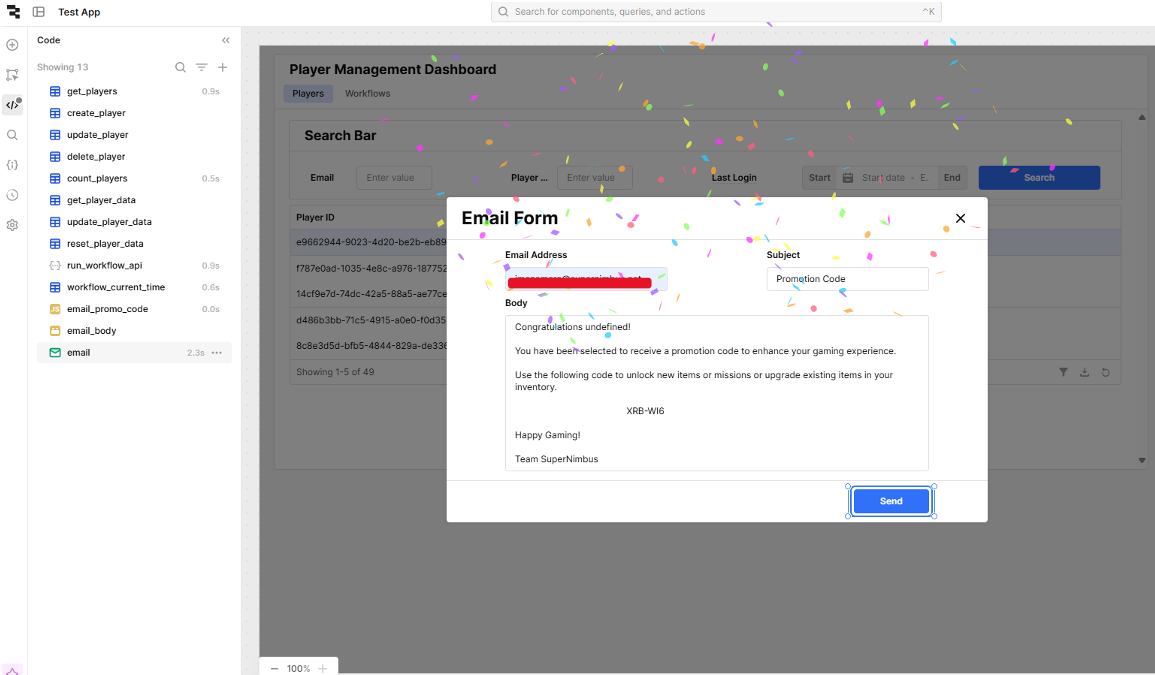
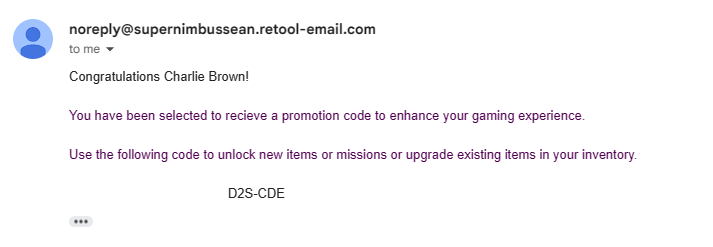
Summary
Now we can send emails to players using the Retool dashboard.
From here we will look at integrating Retool with backend servers.